Commit 09a5131
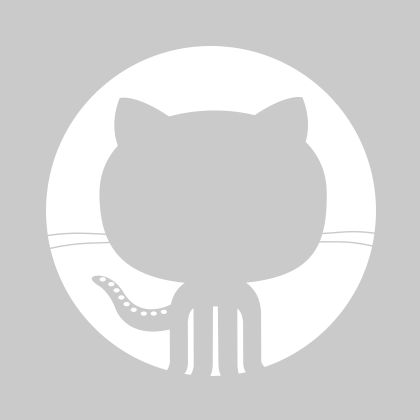
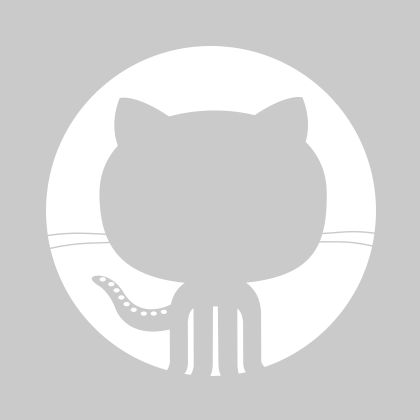
Tim O'Farrell
Tim O'Farrell
1 parent d94b041 commit 09a5131
File tree
24 files changed
+324
-292
lines changed- injecty_config_schemey
- marshy_config_schemey
- schemey
- factory
- json_schema
- schemey_config_default
- tests
- examples
- json_schema
24 files changed
+324
-292
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + |
This file was deleted.
+3-24
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
2 |
| - | |
3 |
| - | |
| 1 | + | |
4 | 2 |
| |
5 |
| - | |
6 |
| - | |
7 | 3 |
| |
8 | 4 |
| |
9 | 5 |
| |
10 |
| - | |
| 6 | + | |
11 | 7 |
| |
12 | 8 |
| |
13 | 9 |
| |
| |||
17 | 13 |
| |
18 | 14 |
| |
19 | 15 |
| |
20 |
| - | |
| 16 | + | |
21 | 17 |
| |
22 | 18 |
| |
23 | 19 |
| |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 | 20 |
| |
42 | 21 |
| |
43 | 22 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
44 | 44 |
| |
45 | 45 |
| |
46 | 46 |
| |
47 |
| - | |
| 47 | + | |
48 | 48 |
| |
49 | 49 |
| |
50 | 50 |
| |
| |||
95 | 95 |
| |
96 | 96 |
| |
97 | 97 |
| |
98 |
| - | |
| 98 | + | |
99 | 99 |
| |
100 | 100 |
| |
101 | 101 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 |
| - | |
5 | 4 |
| |
6 | 5 |
| |
7 | 6 |
| |
| |||
17 | 16 |
| |
18 | 17 |
| |
19 | 18 |
| |
20 |
| - | |
21 | 19 |
| |
22 | 20 |
| |
23 | 21 |
| |
| |||
27 | 25 |
| |
28 | 26 |
| |
29 | 27 |
| |
30 |
| - | |
| 28 | + | |
| 29 | + | |
31 | 30 |
| |
32 | 31 |
| |
33 | 32 |
| |
| |||
55 | 54 |
| |
56 | 55 |
| |
57 | 56 |
| |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
| 9 | + | |
9 | 10 |
| |
10 | 11 |
| |
11 |
| - | |
12 | 12 |
| |
13 |
| - | |
14 |
| - | |
15 |
| - | |
| 13 | + | |
16 | 14 |
| |
17 | 15 |
| |
18 | 16 |
| |
19 | 17 |
| |
20 | 18 |
| |
21 |
| - | |
| 19 | + | |
22 | 20 |
| |
23 | 21 |
| |
24 | 22 |
| |
| |||
30 | 28 |
| |
31 | 29 |
| |
32 | 30 |
| |
33 |
| - | |
| 31 | + | |
34 | 32 |
| |
35 | 33 |
| |
36 | 34 |
| |
37 | 35 |
| |
38 | 36 |
| |
39 | 37 |
| |
40 |
| - | |
41 |
| - | |
42 |
| - |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
| 2 | + | |
2 | 3 |
| |
3 | 4 |
| |
4 | 5 |
| |
| |||
34 | 35 |
| |
35 | 36 |
| |
36 | 37 |
| |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - |
This file was deleted.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + |
0 commit comments