Commit cf3581f
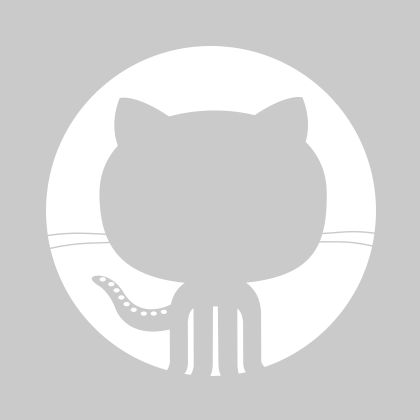
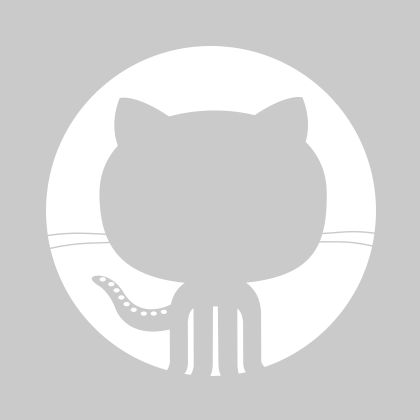
Zhan Milenkov
Zhan Milenkov
1 parent 6630da1 commit cf3581f
File tree
5 files changed
+113
-81
lines changed- src/core
5 files changed
+113
-81
lines changedLines changed: 17 additions & 15 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| 17 | + | |
| 18 | + | |
17 | 19 |
| |
18 | 20 |
| |
19 | 21 |
| |
20 | 22 |
| |
21 | 23 |
| |
22 | 24 |
| |
23 |
| - | |
24 |
| - | |
25 |
| - | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
26 | 28 |
| |
27 | 29 |
| |
28 | 30 |
| |
| |||
43 | 45 |
| |
44 | 46 |
| |
45 | 47 |
| |
46 |
| - | |
47 |
| - | |
48 |
| - | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
49 | 51 |
| |
50 | 52 |
| |
51 | 53 |
| |
| |||
56 | 58 |
| |
57 | 59 |
| |
58 | 60 |
| |
59 |
| - | |
60 |
| - | |
| 61 | + | |
| 62 | + | |
61 | 63 |
| |
62 | 64 |
| |
63 | 65 |
| |
| |||
70 | 72 |
| |
71 | 73 |
| |
72 | 74 |
| |
73 |
| - | |
74 |
| - | |
75 |
| - | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
76 | 78 |
| |
77 | 79 |
| |
78 | 80 |
| |
| |||
85 | 87 |
| |
86 | 88 |
| |
87 | 89 |
| |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
92 | 94 |
| |
93 | 95 |
| |
94 | 96 |
| |
|
Lines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| 17 | + | |
17 | 18 |
| |
18 | 19 |
| |
19 | 20 |
| |
|
Lines changed: 12 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| 17 | + | |
17 | 18 |
| |
18 | 19 |
| |
19 | 20 |
| |
| |||
32 | 33 |
| |
33 | 34 |
| |
34 | 35 |
| |
35 |
| - | |
| 36 | + | |
36 | 37 |
| |
37 | 38 |
| |
38 | 39 |
| |
| |||
305 | 306 |
| |
306 | 307 |
| |
307 | 308 |
| |
308 |
| - | |
| 309 | + | |
309 | 310 |
| |
310 | 311 |
| |
311 | 312 |
| |
| |||
316 | 317 |
| |
317 | 318 |
| |
318 | 319 |
| |
| 320 | + | |
| 321 | + | |
| 322 | + | |
| 323 | + | |
| 324 | + | |
319 | 325 |
| |
320 | 326 |
| |
321 | 327 |
| |
322 | 328 |
| |
323 | 329 |
| |
| 330 | + | |
| 331 | + | |
| 332 | + | |
| 333 | + | |
324 | 334 |
| |
325 | 335 |
| |
326 | 336 |
| |
|
Lines changed: 14 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
| 17 | + | |
17 | 18 |
| |
18 | 19 |
| |
19 | 20 |
| |
| |||
62 | 63 |
| |
63 | 64 |
| |
64 | 65 |
| |
| 66 | + | |
| 67 | + | |
| 68 | + | |
65 | 69 |
| |
66 | 70 |
| |
67 | 71 |
| |
| |||
92 | 96 |
| |
93 | 97 |
| |
94 | 98 |
| |
95 |
| - | |
| 99 | + | |
96 | 100 |
| |
97 | 101 |
| |
98 | 102 |
| |
| |||
160 | 164 |
| |
161 | 165 |
| |
162 | 166 |
| |
163 |
| - | |
| 167 | + | |
164 | 168 |
| |
165 | 169 |
| |
166 | 170 |
| |
| |||
440 | 444 |
| |
441 | 445 |
| |
442 | 446 |
| |
| 447 | + | |
| 448 | + | |
| 449 | + | |
| 450 | + | |
| 451 | + | |
| 452 | + | |
443 | 453 |
| |
444 | 454 |
| |
445 | 455 |
| |
| |||
453 | 463 |
| |
454 | 464 |
| |
455 | 465 |
| |
456 |
| - | |
| 466 | + | |
457 | 467 |
| |
458 | 468 |
| |
459 | 469 |
| |
| |||
519 | 529 |
| |
520 | 530 |
| |
521 | 531 |
| |
522 |
| - | |
| 532 | + | |
523 | 533 |
| |
524 | 534 |
| |
525 | 535 |
| |
|
0 commit comments