Commit 8720b6b
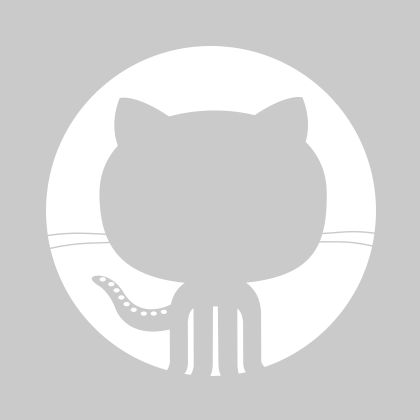
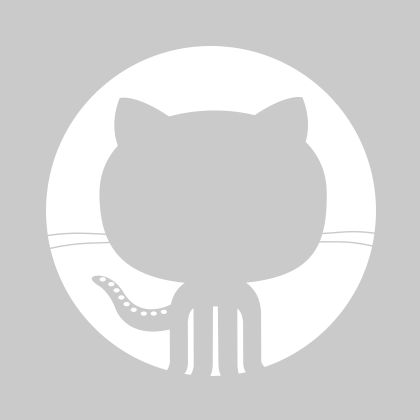
Istvan Neuwirth
Google Java Core Libraries
1 parent 01dcc2e commit 8720b6b
File tree
4 files changed
+86
-4
lines changed- android
- guava/src/com/google/common/primitives
- guava-tests/test/com/google/common/primitives
- guava/src/com/google/common/primitives
- guava-tests/test/com/google/common/primitives
4 files changed
+86
-4
lines changedLines changed: 31 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
198 | 198 |
| |
199 | 199 |
| |
200 | 200 |
| |
| 201 | + | |
| 202 | + | |
| 203 | + | |
| 204 | + | |
| 205 | + | |
| 206 | + | |
| 207 | + | |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
| 212 | + | |
| 213 | + | |
| 214 | + | |
| 215 | + | |
| 216 | + | |
| 217 | + | |
| 218 | + | |
| 219 | + | |
| 220 | + | |
| 221 | + | |
| 222 | + | |
| 223 | + | |
| 224 | + | |
| 225 | + | |
| 226 | + | |
| 227 | + | |
| 228 | + | |
| 229 | + | |
| 230 | + | |
| 231 | + | |
201 | 232 |
| |
202 | 233 |
| |
203 | 234 |
| |
|
Lines changed: 12 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
244 | 244 |
| |
245 | 245 |
| |
246 | 246 |
| |
| 247 | + | |
| 248 | + | |
247 | 249 |
| |
248 | 250 |
| |
249 |
| - | |
| 251 | + | |
250 | 252 |
| |
251 | 253 |
| |
252 | 254 |
| |
253 |
| - | |
| 255 | + | |
254 | 256 |
| |
255 | 257 |
| |
256 | 258 |
| |
| |||
259 | 261 |
| |
260 | 262 |
| |
261 | 263 |
| |
| 264 | + | |
| 265 | + | |
| 266 | + | |
| 267 | + | |
| 268 | + | |
| 269 | + | |
| 270 | + | |
| 271 | + | |
262 | 272 |
| |
263 | 273 |
| |
264 | 274 |
| |
|
Lines changed: 31 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
198 | 198 |
| |
199 | 199 |
| |
200 | 200 |
| |
| 201 | + | |
| 202 | + | |
| 203 | + | |
| 204 | + | |
| 205 | + | |
| 206 | + | |
| 207 | + | |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
| 212 | + | |
| 213 | + | |
| 214 | + | |
| 215 | + | |
| 216 | + | |
| 217 | + | |
| 218 | + | |
| 219 | + | |
| 220 | + | |
| 221 | + | |
| 222 | + | |
| 223 | + | |
| 224 | + | |
| 225 | + | |
| 226 | + | |
| 227 | + | |
| 228 | + | |
| 229 | + | |
| 230 | + | |
| 231 | + | |
201 | 232 |
| |
202 | 233 |
| |
203 | 234 |
| |
|
Lines changed: 12 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
246 | 246 |
| |
247 | 247 |
| |
248 | 248 |
| |
| 249 | + | |
| 250 | + | |
249 | 251 |
| |
250 | 252 |
| |
251 |
| - | |
| 253 | + | |
252 | 254 |
| |
253 | 255 |
| |
254 | 256 |
| |
255 |
| - | |
| 257 | + | |
256 | 258 |
| |
257 | 259 |
| |
258 | 260 |
| |
| |||
261 | 263 |
| |
262 | 264 |
| |
263 | 265 |
| |
| 266 | + | |
| 267 | + | |
| 268 | + | |
| 269 | + | |
| 270 | + | |
| 271 | + | |
| 272 | + | |
| 273 | + | |
264 | 274 |
| |
265 | 275 |
| |
266 | 276 |
| |
|
0 commit comments